LayerMask in Unity are essential for specifying one or more layers on which specific actions can be applied. Think of them as arrays containing layers.
Table of Contents
Using Layers Through the Inspector
You can easily use Layer Masks through Unity’s Inspector:
using UnityEngine;
public class RaycastBehaviour : MonoBehaviour
{
public LayerMask layerMask;
public void RayCast()
{
if (Physics.Raycast(transform.position, transform.forward, out RaycastHit hit, Mathf.Infinity, layerMask))
{
// Actions to be performed with the hit object
}
}
}
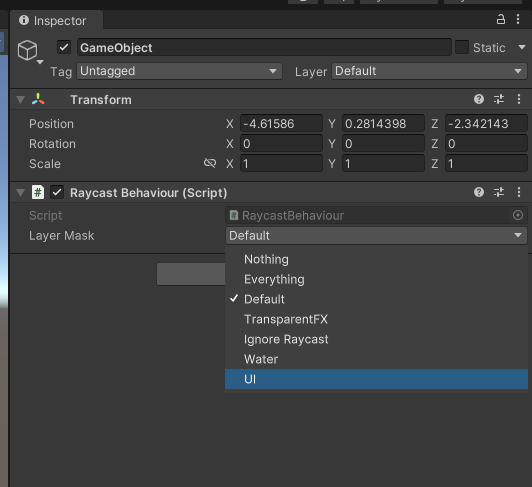
Thanks to Layer Masks, you can easily define which layers will be affected by your actions, such as Raycasting.
Creating a LayerMask in Code
You can also create a LayerMask in code using the static function “GetMask”:
using UnityEngine;
public class RaycastBehaviour : MonoBehaviour
{
public LayerMask layerMask;
private void CreateLayerMask()
{
// Multiple layers
layerMask = LayerMask.GetMask(new string[] { "UI", "Default" });
// Single layer
layerMask = LayerMask.GetMask("UI");
// All except one
layerMask = ~LayerMask.GetMask("UI");
}
}
LayerMask with all layer
If you need a layerMask with all layer:
int allLayers = Physics.AllLayers;
Retrieving the Index of a Layer
If you need to retrieve the index of a layer:
int indexLayer = LayerMask.NameToLayer("UI");
Converting a Layer Index to a LayerMask
You can convert a layer index to a LayerMask:
int indexLayer = LayerMask.NameToLayer("UI");
LayerMask layerMask = 1 << indexLayer;
Retrieving the Name of a Layer Index
If you want to retrieve the name of a layer index:
int indexLayer = LayerMask.NameToLayer("Default");
string nameLayer = LayerMask.LayerToName(indexLayer);
Adding / Removing a Layer via Code
You can add or remove layers via code:
public LayerMask layerMask;
public void AddLayer(int layerIndex)
{
layerMask |= (1 << layerIndex);
}
public void RemoveLayer(int layerIndex)
{
layerMask &= ~(1 << layerIndex);
}
public void Example()
{
AddLayer(LayerMask.NameToLayer("UI"));
}
Checking if a LayerMask Contains a Layer
If you need to know if a LayerMask contains a layer:
public LayerMask layerMask;
public bool Contains(int layerIndex)
{
return layerMask == (layerMask | (1 << layerIndex));
}
public void Example()
{
if (Contains(LayerMask.NameToLayer("UI")))
{
// Do something
}
}
I hope you found this article helpful. Now, you have the knowledge to effectively manage your Layer Masks in Unity.
A word of advice: it would be cleaner to store all functions in a file extension.