Explore in Unity inspector the addition of a button as part of our series on custom inspectors. We’ll also look at how to fully style it and modify its layout.
Adding a Button to the Inspector
To integrate a button into Unity’s Inspector, we’ll utilize the static method GUILayout.Button:
using UnityEditor;
using UnityEngine;
[CustomEditor(typeof(CustomMono))]
public class CustomMonoEditor : Editor
{
public override void OnInspectorGUI()
{
DrawDefaultInspector();
if (GUILayout.Button("My Button"))
{
// Action when the button is clicked
}
}
}
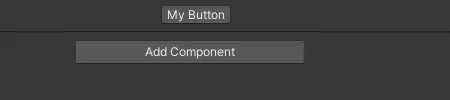
Styling the Button in the Inspector
To stylize our button, we need to pass a GUIStyle as a parameter. The simplest way is to retrieve the base skin and modify it:
var style = new GUIStyle(GUI.skin.button);
style.normal.textColor = new Color32(5, 196, 107, 255); // I like this green
style.fontStyle = FontStyle.Bold;
style.fontSize = 35;
if (GUILayout.Button("My Button", style))
{
// Action when the button is clicked
}
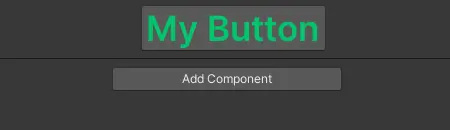
Similar to its UI counterpart, you can change the button’s color for different states: normal, hover, and active. For further customization, refer to the article on customizing GUI background colors.
Styling the Layout of the Button
A general rule applying to all elements created from the GUILayout class is the ability to pass a GUILayoutOption array. It can contain various options such as:
if (GUILayout.Button("My Button", new GUILayoutOption[] { GUILayout.MaxWidth(250) }))
{
// Action when the button is clicked
}
GUILayout.Button Or GUI.Button
Both functions perform the same task; however, GUI requires an absolute position, while GUILayout relies on the last created element for placement. Examining Unity’s source code reveals that most GUILayout functions internally call their GUI counterparts using GUILayoutUtility.GetRect for positioning.
I hope this guide has provided additional insights into custom editors, specifically focusing on Unity inspector button.
Don’t forget to use custom Editors, they empower developers to tailor the Unity Editor to their specific needs, enhancing workflow efficiency and providing a more intuitive and streamlined development experience.
Thank you for reading, happy coding!
Original image from Mohamed Hassan