Unity Distance between 2 points
In a Unity 3D context, the distance between two points is obtained through the method Vector3.Distance
.
The returned value is always a positive float, possibly equal to zero.
using UnityEngine;
public class DistanceMonobehaviour : MonoBehaviour
{
public void DistanceByPoint(Vector3 point)
{
float distance = Vector3.Distance(transform.position, point);
}
Note that distance can be converted to int.
However, in a Unity 2D context, the distance is calculated using the Vector2.Distance
method.
using UnityEngine;
public class DistanceMonobehaviour : MonoBehaviour
{
private void Start()
{
Vector2 a = new Vector2(0f, 5f);
Vector2 b = new Vector2(1f, 2f);
float distance = Vector2.Distance(a, b);
}
How Distance Works Mathematically?
To continue learning, it’s always interesting to know how the distance function works under the hood.
Then, if we go back to our mathematics lessons, the formula used to calculate the distance between two points is as follows: d=√((b.x-a.x)²+(b.y-a.y)²)
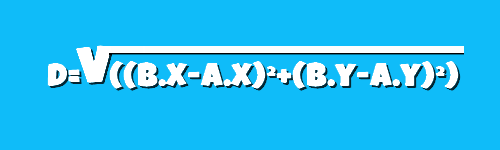
To assist us in our Unity calculations, we have access to the methods:
Here is its application in C#:
public float DistanceByMath(Vector2 a, Vector2 b)
{
float magnitudeX = Mathf.Pow(b.x - a.x, 2f);
float magnitudeY = Mathf.Pow(b.y - a.y, 2f);
return Mathf.Sqrt(magnitudeX + magnitudeY);
}
Get Distance with magnitude in Unity
To continue in a more Unity-centric context, you can also calculate the distance by subtracting the two vectors and then obtaining their magnitude.
PS: Magnitude is the length of the vector represented by √(x²+y²).
If we take our specification with (a) and (b), we then get (a – b).magnitude:
This results in a more concise code than the purely mathematical approach:
public float DistanceUnity(Vector2 a, Vector2 b)
{
var subtract = a - b;
return subtract.magnitude;
}
Conclusion
Even though it is always interesting to know the different ways to calculate distance in Unity, the static methods of Vector3 and Vector2 remain preferable for this task due to their simplicity of execution.
In conclusion, there is no need to reinvent the wheel, Unity already has the right functions.