In Unity, play animation can be achieved in three distinct ways, each offering its unique utility.
Regardless of the method chosen, you will require an Animator component, containing your animation states.
Table of contents
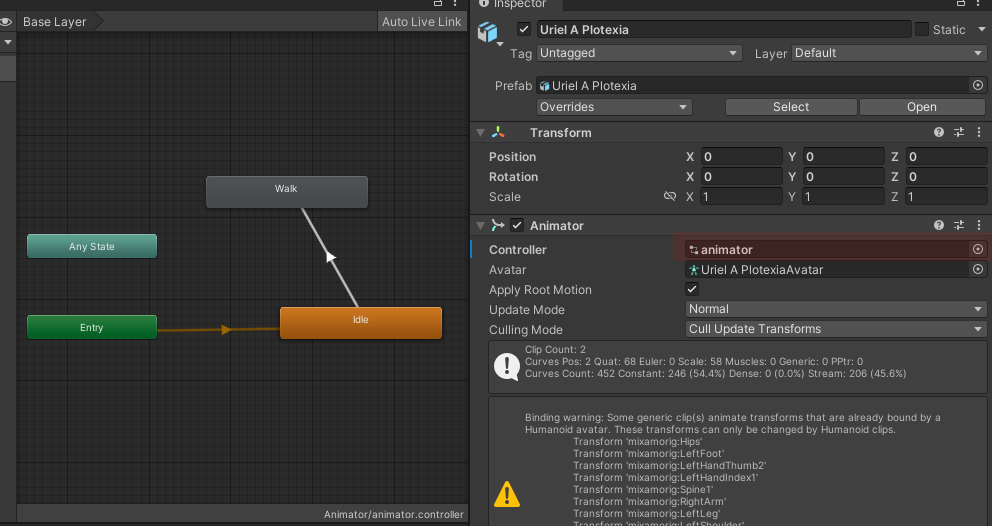
How to play Animation Without Parameters
To play an animation in the code, we use the “Play” property in our “Animator“:
using UnityEngine;
public class AnimatorTest : MonoBehaviour
{
public Animator animator;
private int idleHash;
private void Awake()
{
idleHash = Animator.StringToHash("Idle");
}
public void Start()
{
animator.Play(idleHash);
}
}
This approach is particularly advantageous when you desire full programmatic control over your animations.
The usage of Animator.StringToHash improves performance, as it precomputes a hash of the animation state name.
Although this optimization may not significantly impact on a single call, it becomes essential in scenarios with numerous state changes, such as within an “Update” loop.
Using Bool Parameters
- Open the Animator tab.
- Create a boolean parameter named “isWalk”
- Establish a transition from the “Idle” state to the “Walk” state.
- Set the condition “isWalk” to true.
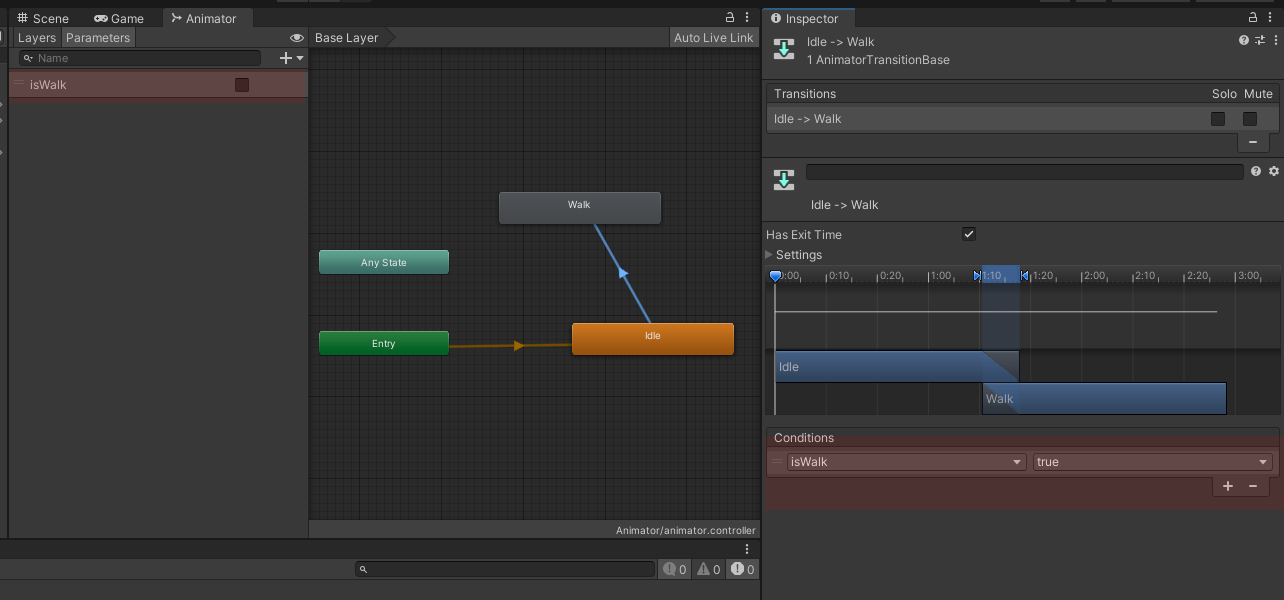
Then, to play the animation, we use the “SetBool” property to set our boolean to true.
using UnityEngine;
public class AnimatorTest : MonoBehaviour
{
public Animator animator;
private int _isWalkHash;
private void Awake()
{
_isWalkHash = Animator.StringToHash("isWalk");
}
public void Start()
{
animator.SetBool(_isWalkHash, true);
}
}
Employing boolean parameters is particularly beneficial when you wish to initiate an animation when a boolean becomes true and halt it when the boolean becomes false.
This method is ideally suited for animations like character walking cycles.
Utilizing Trigger Parameters
- Access the Animator tab.
- Generate a trigger parameter named “isWalk”
- Define a transition from the “Idle” state to the “Walk” state.
- Implement a transition condition based on the trigger “isWalk”
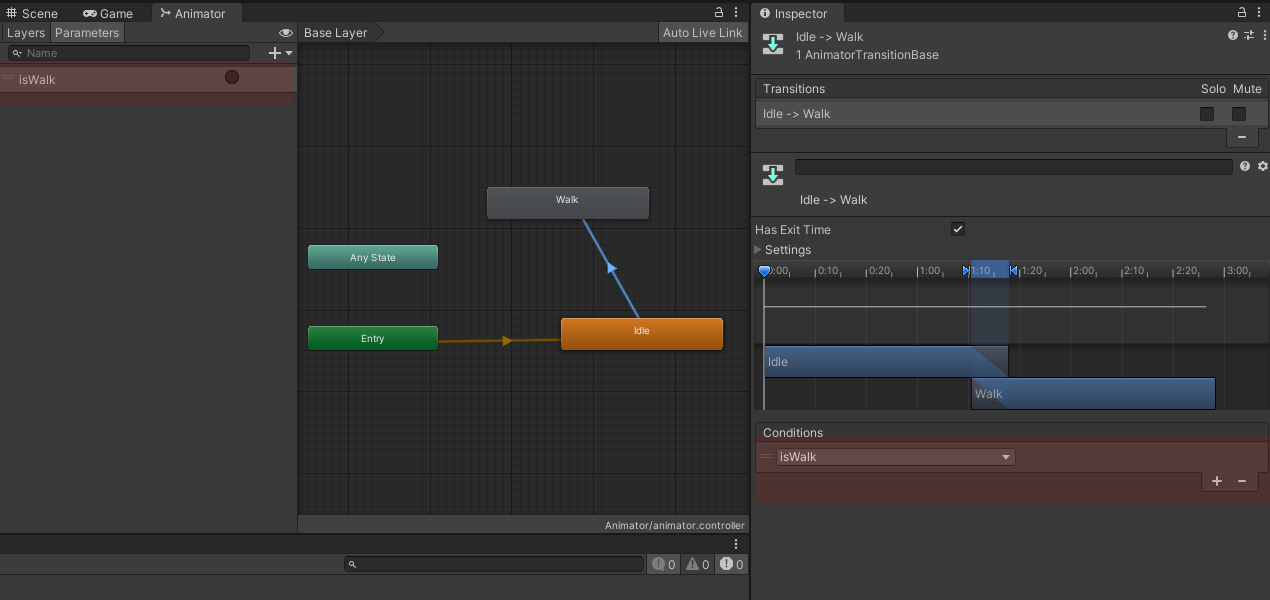
Next, to play the animation with our trigger, this time we use the “SetTrigger” property to activate our trigger.
using UnityEngine;
public class AnimatorTest : MonoBehaviour
{
public Animator animator;
private int _isWalkHash;
private void Awake()
{
_isWalkHash = Animator.StringToHash("isWalk");
}
public void Start()
{
animator.SetTrigger(_isWalkHash);
}
}
Trigger parameters are ideal for animations such as attack sequences, where you want an animation to play once and automatically exit.
When a trigger is set, it automatically becomes unset upon the animator’s state change.
If you need to reset a trigger for concurrent execution, you can call the “ResetTrigger” function. This method ensures that animations do not get interrupted during execution.
Play animation on keypress
To play an animation when a key is pressed, we can use the Input Manager and its “Input.GetKey” method:
using UnityEngine;
public class AnimatorTest : MonoBehaviour
{
public Animator animator;
private int idleHash;
private void Awake()
{
idleHash = Animator.StringToHash("Idle");
}
public void Update()
{
if (Input.GetKey(KeyCode.A))
{
// Key A is held
animator.Play(idleHash);
}
}
}
As a reminder, it may now be preferable to use Input System’s new Input Manager instead.
Play animation in reverse
To play the animation in reverse, we can modify our state’s “speed” parameter in the animator and give it a negative value.
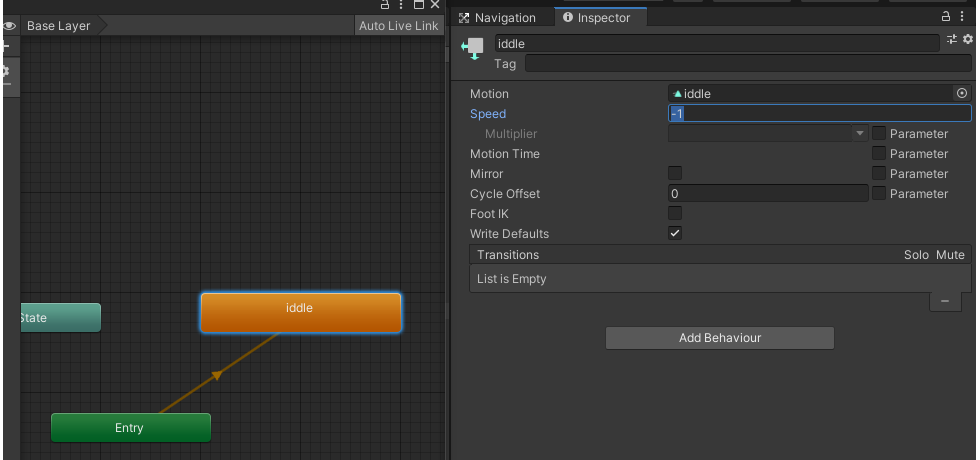
If you’d rather duplicate your animation and turn it into a reverse, I suggest you take a look at this post.
Bravo, what phrase…, a remarkable idea
The excellent answer, gallantly 🙂